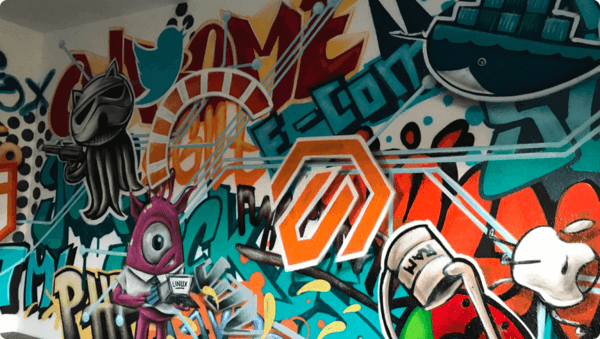
Animations
This article provides an overview of the fundamental principles of developing animations for websites from the standpoint of a front-end developer. We'll look at the many types of animations used in web development, compare implementation approaches, examine which animations should be avoided due to performance implications, and focus on how to utilize animations appropriately to create a great user experience. In addition, we'll go over expectations for UX designers during the animation development process to guarantee successful collaboration and
high-quality results.
Main animation types
- Transforms: these are manipulations of elements that change their size, rotation, offset, or skew. You can change the appearance of an element without affecting the document's flow by using properties like as
transform: scale()
,transform: rotate()
,transform: translate()
. These animations are typically used to create scaling, rotation, or movement effects for elements on
the webpage. - Transitions: these are smooth transitions between different states of CSS properties of elements that are activated when these properties change. For example, by setting, you can make a smooth flow from one state of an element to another when changing properties such as color, size, transparency, and so on. This type of animation is useful for creating hover effects or active button states.
- Keyframe animations are more complicated and controlled, with multiple steps, looping behavior, and the ability to respond to particular triggers. Animations can be created using
@keyframes
, with each step representing an element's intermediate state. This type of animation allows you to create long-lasting effects, such as smoothly moving elements across the screen or changing their color at certain intervals.
There are several ways to implement animations: from using just CSS to more intricate methods involving JavaScript libraries and frameworks. Depending on the project's requirements, the tools used to create animations might have a considerable impact on the final product. In addition to traditional CSS features, modern tools such as Alpine.js and TailwindCSS make it easy to create interactive and dynamic websites, drastically simplifying the animation process. Let us look at how these tools can help improve the implementation of animations on websites.
Alpine.js + TailwindCSS
Alpine.js and TailwindCSS are excellent tools for creating animations with little coding. Alpine.js handles reactivity and state management, whereas TailwindCSS takes a more utilitarian approach to style, allowing you to add animations to elements easily.
Advantages of using Alpine.js + TailwindCSS:
Easy integration of animations without writing any extra JavaScript code. Convenient class management with TailwindCSS allows you to customize styles and animations quickly.
The reactivity of Alpine.js allows you to easily launch animations depending on various events, for example when an element enters the viewport using x-intersect
.
Animation code example using Alpine.js + TailwindCSS:
<div x-data="{ 'animationTriggered': false }"
:class="{'fade-in': animationTriggered}"
x-intersect:enter.once="animationTriggered = true"
class="opacity-0 transition-opacity duration-700">
This text will appear with a fade-in effect when you scroll
</div>
<style>
.fade-in {
opacity: 1;
}
</style>
Code example of the same animation in pure CSS:
<div class="fade-in-element">
This text will appear with a fade-in effect when you scroll
</div>
<style>
.fade-in-element {
opacity: 0;
transition: opacity 0.7s ease;
}
.fade-in-element.visible {
opacity: 1;
}
</style>
<script>
document.addEventListener('scroll', function() {
const element = document.querySelector('.fade-in-element');
const rect = element.getBoundingClientRect();
if (rect.top <= window.innerHeight && rect.bottom >= 0) {
element.classList.add('visible');
}
});
</script>
Animations to avoid and performance optimization
Animations to avoid or use with caution:
- Animations on properties that cause repaint: Animations that change properties such as width, height, margin, and padding may force the entire document to be repainted, putting an enormous load on the browser. For example, animations that resize elements cause the browser to recalculate all other elements on the page, leading to delays or freezes.
- Animations on properties that cause a reflow: Properties that influence the arrangement of other elements on the page, such as top, left, right and bottom, cause a layout to be recalculated. This is an even more costly process for the browser than redrawing and can have a significant impact on performance, particularly for pages with a large number of elements.
- Large or complex animations: animations that span numerous elements or require massive calculations might severely slow down your website. For example, animations that influence multiple items at the same time or use complex mathematical formulas for operations might impose a strain on the CPU
Recommendations for improving performance when using CSS animations:
- Animate properties that have no impact on the layout: a best practice is to animate properties that do not require layout recalculation or redrawing. The properties include transform and opacity. Animations with these properties are performed at the hardware level (via the GPU), making them more efficient.
- Optimize the duration and number of animations: Only use animations when truly necessary and keep their duration to a minimum. Shorter animations (0.30.5 seconds) are usually perceived more naturally and put a smaller load on the system. Also, limit the number of animations that can be run simultaneously.
- Use
will-change
with caution: Thewill-change
property informs the browser about which elements will change, allowing it to optimize their processing. However, use with caution, as excessive use can result in performance loss
caused by memory overflow. - Avoid using animations at key navigation points: avoid utilizing animations at important navigation moments. Avoid using complex or lengthy animations for website load times, page transitions, or other key actions. They can reduce system responsiveness and affect the user experience.
Following these recommendations will help to maintain a balance between the attractiveness of animations and the site's speed, resulting in a comfortable user experience.
Basic principles of high-quality animation: meeting user expectations
High-quality animations on a website not only catch the eye, but they also improve the user experience by making interaction with the interface easier to understand. Certain standards must be followed to ensure that animations do not distract or cause discomfort to the user.
Principles of high-quality animation:
- Unobtrusiveness and appropriateness: animation should support the overall context of the page without distracting from the main content. It should be used only where it is really necessary, for example, to emphasize important
user actions or to provide visual feedback. - Consistency: every animation on a website should have a similar style. This generates a consistent impression and makes it easier for people to explore the website. For example, if one element shows smoothly on the screen, other related items should also have a smooth animation.
- Meet user expectations: animations should be predictable. For example, when users click on a button, they expect a specific action, which the animation should clearly represent. If the animation does not meet expectations, it can cause confusion or frustration.
- Speed and duration: the animation's duration should be balanced: it should not be too rapid so that the user misses essential information, nor should it be too long to delay the user. The ideal length for most animations is 300-500 milliseconds.
- Performance: a high-quality animation should be computationally light, preventing page slowness or freezes. Use animations with a low impact on performance and optimize your code to ensure that your animations run smoothly even on less powerful devices.
- Animations of background elements: animations of background elements, such as images or gradients, can add depth to a website. However, they should be used with caution to not to overload the user. For example, a steady movement of a background image or a color change in a gradient might create a depth effect, but too fast or bright changes can be distracting or even annoying.
- Timing functions:
e ase-in
andease-out
make animations more natural since they imitate real-world processes. This helps the user feel more comfortable and minimizes cognitive load.Linear
may give an artificial sensation and
should only be used when a consistent property change is necessary, such as in cyclic animations. Choosing the proper timing function can improve the user experience by making animations easier to understand.
Collaboration with a UX designer for high-quality animations
To successfully create animations on a website, a UX designer and a frontend developer must work closely together. As a front-end developer, I have specific expectations for the designer that will help make the animation creation process more efficient and successful.
A clear understanding of the purpose of the animation
Every animation should serve a specific purpose, such as improving interaction, drawing attention to a particular feature, or providing user feedback. It is critical that the designer understands what function the animation should serve and can explain this to the developer.
Detailed and clear specifications
The designer should provide precise information about the animations, such as duration, delay and final result. The specs should contain animation samples or mockups to help the developer correctly reproduce the idea.
Consistency in the use of animations
All animations on the site must be consistent in style and rhythm. This results in a more comprehensive user experience and eliminates visual inconsistencies. The designer should create guidelines or a library of animations for use during development.
Flexibility and willingness to change
Due to technical constraints or performance limitations, not all design concepts are easily implemented. The designer must be open to debating and changing their decisions as needed. This will guarantee that the animations are implemented successfully while maintaining performance and quality.
Prototyping and testing
If possible, the designer should create animated prototypes. This will help to evaluate at an early stage how the animation fits into the entire interface and whether it meets user expectations. Prototypes help to avoid unexpected problems at the development stage. Providing detailed instructions and examples, as well as being open to developer feedback, will guarantee that animations are both user-friendly and technically advanced.
Final review
Website animations are more than simply decoration; they are a powerful tool that can dramatically improve the user experience. Properly designed animations contribute to a website's intuitiveness, attractiveness, and usability. However, it is vital to note that animations should not only be visually appealing, but also work well, match user expectations, and be appropriate for their intended usage.